de.softpro.signware.SPCompare Class Reference
Inheritance diagram for de.softpro.signware.SPCompare:
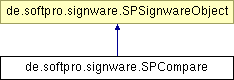
Detailed Description
Compare a SPSignature or SPImage object against a SPReference or SPTemplate or SPImage object.
SPCompare instances save all properties such as match quality or projection factor within the instance object for later usage in the compare functions.
The application will typically select the engines to use (setMode), set compare parameters (setMatchQualityEx) and perform the comparison (compareReference, compareTemplate, compareImage).
The final result (getResult) is composed from the result of all engines, the final result will be false (no match) if any of the selected engines calculated a match rate less than the minimum match quality for the engine.
The application may query the detailed results of all selected engines (getDetailResult, getNumResults) and implement another match algorithm based on the detail results.
The SPCompare class uses several engines to compare signatures, a dynamic, a static and a statistic engine. The match quality of all engines is normalized in the range 0 ... 100, where 0 means very low match quality, and 100 means high probability that signature and reference were entered by the same person.
Do not assume that there is a linear relation of match quality and signature similarity.
Please read section sec_Performance and section sec_StaticPerformance
- Operating Systems:
- Windows (Win32)
// Example (Java) class MyCompare { public int compare(SPReference aRef, SPSignature aSig, int iMode) throws SPSignwareException { SPCompare spCompare = new SPCompare(); if((iMode & SPCompare.SP_COMPARE_DYNAMIC) != 0) { int iSampleRateSig = aSig.getSampleRate(); int iPressSig = aSig.getMaxPressure(); SPSignature sig0 = new SPSignature(sRef, 0); int iSampleRateRef = sig0.getSampleRate(); int iPressRef = sig0.getMaxPressure(); int iNumSignos = aRef.getSignatureCount(); if(iPressSig <= 2 || iPressRef <= 2 || iSampleRateSig == 0 || iSampleRateRef == 0 || iNumSignos < 2) { iMode &= ~SPCompare.SP_COMPARE_DYNAMIC; iMode |= SPCompare.SP_COMPARE_DSTAT; } } spCompare.setMode(iMode); spCompare.compareReference(aRef, aSig); // Dump detailed results getDetailResult(spCompare, SPCompare.SP_COMPARE_STATIC_ENGINE, "static"); getDetailResult(spCompare, SPCompare.SP_COMPARE_DYNAMIC_ENGINE, "dynamic"); getDetailResult(spCompare, SPCompare.SP_COMPARE_DSTAT_ENGINE, "statistic"); // Return the final result as computed by the SPCompare object return spCompare.getResult(); } private void getDetailResult(SPCompare spCompare, int iEngine, String strEngine) { String strRes = ""; try { int iNumResults = spCompare.getNumResults(iEngine); if(iNumResults > 0) { for(int ix = 0; ix < iNumResults; ix++) { int iMatch = spCompare.getDetailResult(iEngine, ix); if(iMatch < 0) { // an error occured strRes += strEngine + "[" + ix + "]: " + iMatch + ", " + SPSignware.getErrorString(iMatch) + "\n"; } else { strRes += strEngine + "[" + ix + "]: " + iMatch + "\n"; } } } } catch (SPSignwareException se) { System.out.println("getDetailResult() - " + se.getMessage()); } System.out.println(strRes); } }
Public Member Functions | |
void | compareImage (SPImage pImage, SPImage pSignature) throws SPSignwareException |
compare a signature with an Image | |
void | compareImage (SPImage pImage, SPSignature pSignature) throws SPSignwareException |
compare a signature with an Image | |
void | compareReference (SPReference pReference, SPImage pImage) throws SPSignwareException |
compare a signature with a reference | |
void | compareReference (SPReference pReference, SPSignature pSignature) throws SPSignwareException |
compare a signature with a reference | |
void | compareTemplate (SPTemplate pTemplate, SPImage pImage) throws SPSignwareException |
compare a signature with a template | |
void | compareTemplate (SPTemplate pTemplate, SPSignature pSignature) throws SPSignwareException |
compare a signature with a template | |
int | getDetailResult (int iEngine, int iIndex) throws SPSignwareException |
Get the result for the specified engine. | |
int | getDynamicMatchQuality () throws SPSignwareException |
Get the min. Match rate for dynamic verfication. | |
int | getDynamicResult () throws SPSignwareException |
Get the result of a dynamic comparison. | |
int | getMatchQualityEx (int iEngine) throws SPSignwareException |
Get the min. Match rate for the specified engine. | |
int | getMode () throws SPSignwareException |
Get the compare mode. | |
int | getNumResults (int iEngine) throws SPSignwareException |
Get the number of available results for the specified engine. | |
int | getNumStaticResults () throws SPSignwareException |
Query the number of statics results available. | |
int | getResult () throws SPSignwareException |
Get the final result of a comparison. | |
int | getStaticMatchQuality () throws SPSignwareException |
Get the min. Match rate for static verfication. | |
int | getStaticResult (int iIndex) throws SPSignwareException |
Get the result of a static comparison. | |
void | setDynamicMatchQuality (int iMinDynamicQuality) throws SPSignwareException |
Set the min. Match rate for sdynamic verfication. | |
void | setMatchQuality (int iMinDynamicQuality, int iMinStaticQuality) throws SPSignwareException |
Set the min. Match rate for static and dynamic verfication. | |
void | setMatchQualityEx (int iEngine, int iMatchRate) throws SPSignwareException |
Set the min. Match rate for the specified engine. | |
void | setMaximumResolution (int iMaximumResolution) throws SPSignwareException |
Set the maximum Resolution for signature and reference which are passed to the engines. | |
void | setMode (int iMode) throws SPSignwareException |
Set the compare mode. | |
void | setStaticMatchQuality (int iMinStaticQuality) throws SPSignwareException |
Set the min. Match rate for static verfication. | |
void | setTicket (SPTicket spTicket) throws SPSignwareException |
Pass a license for one or more compares or SPTicket.ticket_compare_dynamic). | |
SPCompare () throws SPSignwareException | |
Create a Compare data structure. | |
Static Public Attributes | |
static final int | SP_COMPARE_AUTO_ENGINE = 0x80 |
Flag to use all engines available for the according compare objects. | |
static final int | SP_COMPARE_AUTOZOOM = 0x20 |
Flag to size the projected signature / reference images for compares. | |
static final int | SP_COMPARE_DESKEW = 4 |
Flag to deskew signature and reference / template. | |
static final int | SP_COMPARE_DSTAT = 0x40 |
Flag to include a dstat (statistic) comparison. | |
static final int | SP_COMPARE_DSTAT_ENGINE = 1 |
Engine specifyer: use dstat (statistic) engine. | |
static final int | SP_COMPARE_DYNAMIC = 2 |
Flag to include a dynamic comparison. | |
static final int | SP_COMPARE_DYNAMIC_ENGINE = 2 |
Engine specifyer: use dynamic (adsv) engine. | |
static final int | SP_COMPARE_MIRROR = 0x10 |
Flag to compare signatures and references / templates that are written from right to left. | |
static final int | SP_COMPARE_NORMALIZE_SAMPLERATE = 8 |
Flag to normalize the sample rate of signature and reference or template. | |
static final int | SP_COMPARE_STATIC = 1 |
Flag to include a static comparison. | |
static final int | SP_COMPARE_STATIC_ENGINE = 3 |
Engine specifyer: use static (sival) engine. | |
Package Functions | |
final native int | jniFree () |
Constructor & Destructor Documentation
|
Create a Compare data structure. The default mode is set to SP_COMPARE_STATIC | SP_COMPARE_DYNAMIC | SP_COMPARE_DESKEW.
|
Member Function Documentation
|
compare a signature with an Image
|
|
compare a signature with an Image
|
|
compare a signature with a reference
|
|
compare a signature with a reference
|
|
compare a signature with a template
|
|
compare a signature with a template
|
|
Get the result for the specified engine.
|
|
Get the min. Match rate for dynamic verfication.
|
|
Get the result of a dynamic comparison.
|
|
Get the min. Match rate for the specified engine.
|
|
Get the compare mode.
|
|
Get the number of available results for the specified engine.
|
|
Query the number of statics results available.
|
|
Get the final result of a comparison.
|
|
Get the min. Match rate for static verfication.
|
|
Get the result of a static comparison. The threshold for a match is typically set at a matchrate of 80.
Static comparison is called for each signature of the reference. The result of each comparison is accessible via parameter iIndex. Please pass iIndex = 0 for the first comparison.
|
|
Implements de.softpro.signware.SPSignwareObject. |
|
Set the min. Match rate for sdynamic verfication. Default valus for dynamic quality rates are 80. Values must be within 0 and 100.
|
|
Set the min. Match rate for static and dynamic verfication. Default values for static and dynamic quality rates are 80. Values must be within 0 and 100.
|
|
Set the min. Match rate for the specified engine.
|
|
Set the maximum Resolution for signature and reference which are passed to the engines. The default maximum resolution is 300 DPI. This parameter will be ignored if the flag SP_COMPARE_AUTOZOOM is not set If maximum resolution is set to 0 and flag SP_COMPARE_AUTOZOOM is set, then signature and reference will be resized to the lower resolution of signature and reference.
|
|
Set the compare mode. The default mode is SP_COMPARE_DESKEW | SP_COMPARE_AUTOZOOM.
|
|
Set the min. Match rate for static verfication. Default valus for static quality rates is 80. Values must be within 0 and 100. *
|
|
Pass a license for one or more compares or SPTicket.ticket_compare_dynamic). The ticket must have been charged for usage SPTicket.ticket_compare_static or SPTicket.ticket_compare_dynamic.
|
Member Data Documentation
|
Flag to use all engines available for the according compare objects.
The compare functions will do a at least static comparison with the reference. |
|
Flag to size the projected signature / reference images for compares. The signature and reference will be resized to the lowest resolution value of signaure, reference and MaxResolution.
|
|
Flag to deskew signature and reference / template.
The SPCompare-functions deskew signature and reference / template before calling a static comparison. |
|
Flag to include a dstat (statistic) comparison. The SPCompare-function will do a dstat comparison with the reference. |
|
Engine specifyer: use dstat (statistic) engine.
|
|
Flag to include a dynamic comparison. The SPCompare-function will do a dynamic comparison with the reference or the template. |
|
Engine specifyer: use dynamic (adsv) engine.
|
|
Flag to compare signatures and references / templates that are written from right to left.
Some nations write from right to left. This flag will optimize the static signature compare engine for signatures that were entered writing from left to right. The SPCompare-functions will mirror signature and reference / template before calling a static comparison. |
|
Flag to normalize the sample rate of signature and reference or template.
The SPCompare-functions normalize the tablet sample rate of signature and reference or template before calling a static comparison. |
|
Flag to include a static comparison. The SPCompare-function will do a static comparison of the signature with all reference signatures, or the template. |
|
Engine specifyer: use static (sival) engine.
|
The documentation for this class was generated from the following file: